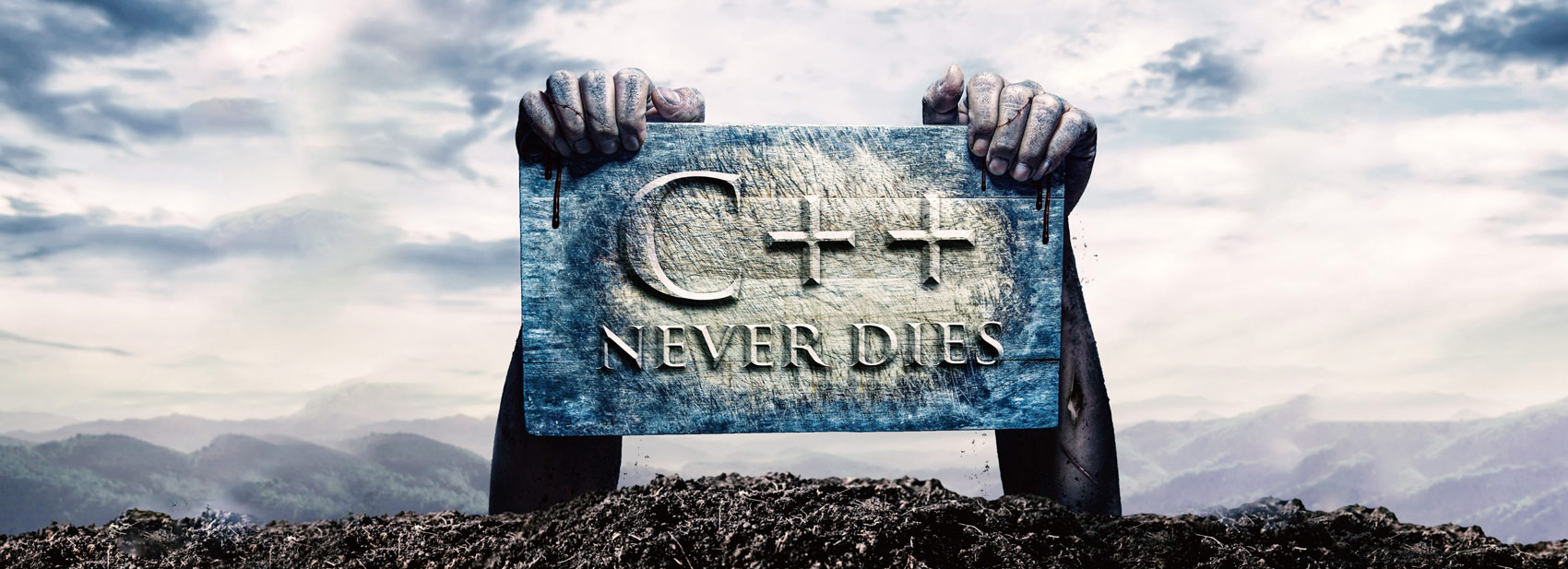
Motivation
1.unique_ptr 独享内存资源所有权的指针
2.unique_ptr 实现资源所有权转移
unique_ptr 独享内存资源所有权的指针
When ownership of resource is required. When we want single or exclusive ownership of a resource, then we should go for unique pointers. Only one unique pointer can point to one resource. So, one unique pointer cannot be copied to another. Also, it facilitates automatic cleanup when dynamically allocated objects go out of scope and helps preventing memory leaks.
intuitively,
1.当我们采用 unique_ptr 时, 会在堆上为数据类型 A 的示例分配内存, 并且用 ptr1 指向 object. ptr1 是 object A 的唯一所有者, 它管理该 object 的生命周期, 如果 ptr1 被重置或者超出 scope 时, 内存自动释放并且 A 被销毁.
2.我们想完全唯一化控制内存资源所有权的时候, 我们采用 unique_ptr. 在使用 unique_ptr时, 内存资源所有权只能发生 [移动], 而不能复制或者拷贝. 比如完成转移之后, 原有的指针会变成空指针.
unique_ptr 实现资源所有权转移
#include <memory>
#include <iostream>
using namespace std;
int main () {
unique_ptr<int> p(new int(5));
cout << "*p=" << *p << ", p.get()=" << p.get() <<endl;
// unique_ptr<int> p2(p); // 报错, unique_ptr 不支持拷贝和赋值
// unique_ptr<int> p3 = p; // 报错, unique_ptr 不支持拷贝和赋值
unique_ptr<int> p1 = std::move(p); // 通过移动语义实现了地址拷贝
cout << "*p1=" << *p1 << ", p1.get()=" << p1.get() <<endl;
// 这时候 p 变成了空指针
if (p == nullptr) {
cout << "p is nullptr now" << endl;
} else {
cout << "p.get()=" << p.get() <<endl;
}
return 0;
}
Reference
1.Unique_ptr in C++. https://www.geeksforgeeks.org/unique_ptr-in-cpp/.
2.C++ 11 创建和使用 unique_ptr. https://www.cnblogs.com/DswCnblog/p/5628195.html.
转载请注明来源 goldandrabbit.github.io