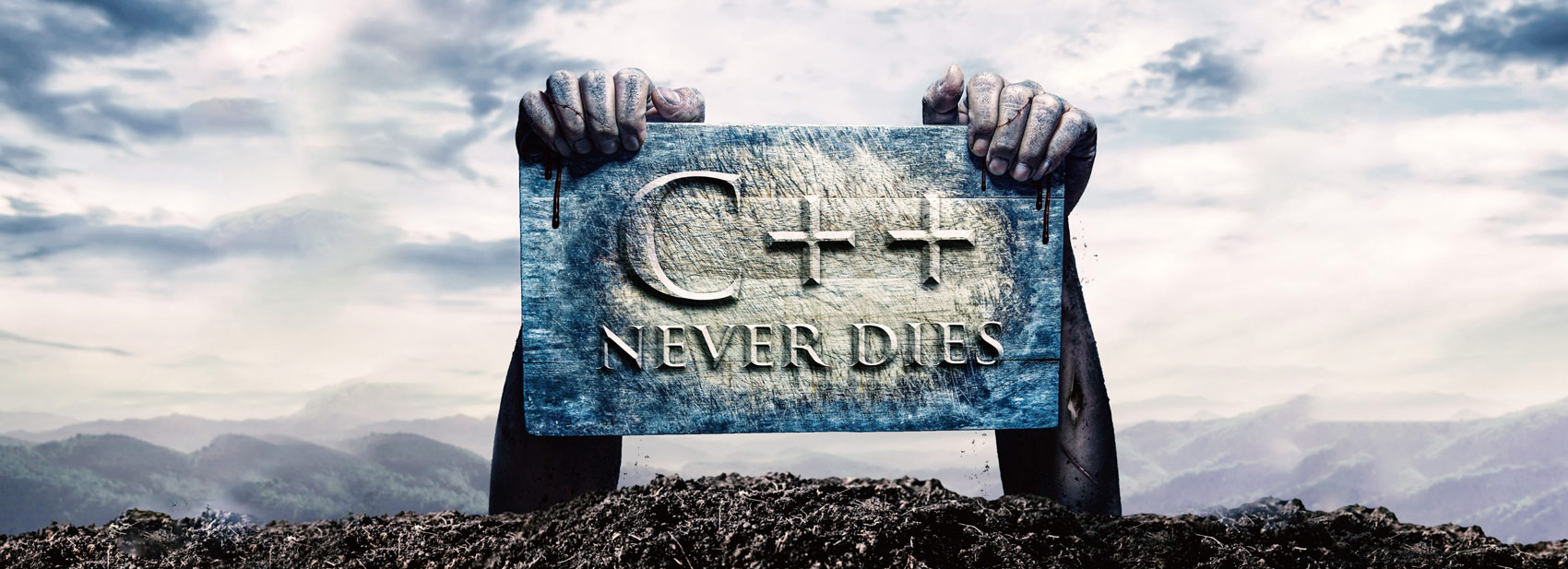
Overview
1.Header file 的作用
2.Header file Best Practices 头文件最佳实践
Header file 的作用
为什么不直接引入 .cc(.cpp) 文件?
circle.h
#ifndef CIRCLE_H
#define CIRCLE_H
class Circle {
private:
double r;
public:
Circle();
Circle(double R);
double calcArea();
};
#endif
circle.cc
#include <iostream>
#include "circle.h"
Circle::Circle() {
this->r = 5.0;
}
Circle::Circle(double R) {
this->r = R;
}
double Circle::calcArea() {
return 3.14 * r * r;
}
main.cc
#include <iostream>
#include "circle.h"
int main() {
Circle c(3);
std::cout << "area of circle is " << c.calcArea() << std::endl;
return 0;
}
Header file Best Practices 头文件最佳实践
Always include header guards (we’ll cover these next lesson).
Do not define variables and functions in header files.
Give a header file the same name as the source file it’s associated with (e.g. grades.h is paired with grades.cpp).
Each header file should have a specific job, and be as independent as possible. For example, you might put all your declarations related to functionality A in A.h and all your declarations related to functionality B in B.h. That way if you only care about A later, you can just include A.h and not get any of the stuff related to B.
Be mindful of which headers you need to explicitly include for the functionality that you are using in your code files.
Every header you write should compile on its own (it should #include every dependency it needs).
Only #include what you need (don’t include everything just because you can).
Do not #include .cpp files.
Prefer putting documentation on what something does or how to use it in the header. It’s more likely to be seen there. Documentation describing how something works should remain in the source files.
Reference
[1]. https://www.learncpp.com/cpp-tutorial/header-files/
转载请注明来源 goldandrabbit.github.io